Javascript
Richard Wong 20 October, 2008
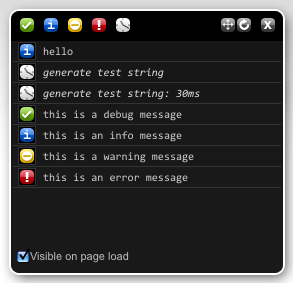
Everyone who programmed in Javascript will know that one of the most common way to debug across browsers is the alert() function. Although, you can use tools like Firebug and their console api to output. It doesn’t work on IE or Safari. So a pure Javascript based solution would be great.
Blackbird is one of the latest script that does just that. It offers:
a dead-simple way to log messages in JavaScript and an attractive console to view and filter them. You might never use alert()
again.
All you need to do is include the Blackbird script and style. Then you can start logging messages using their APIs including different message types and profiling as shown below:
-
log.debug( message )
- Add a debug message to Blackbird
message
: the string content of the debug message
log.info( message )
- Add an info message to Blackbird
message
: the string content of the info message
log.warn( message )
- Add a warning message to Blackbird
message
: the string content of the warn message
log.error( message )
- Add an error message to Blackbird
message
: the string content of the warn message
log.profile( label )
- Start/end a time profiler for Blackbird. If a profiler named
string
does not exist, create a new profiler. Otherwise, stop the profiler string
and display the time elapsed (in ms).
Blackbird
Richard Wong 21 September, 2008
Charts are important in visualising data to give a more profound understanding of the nature of given problem. A good charting solution for should render charts dynamically from raw data and allow multiple type of data presentation.
Traditionally we generate charts on the server-side but that means it will take up more bandwidth by the image all the way across the internet. Ideally we would just send the data to the browser and it will render the chart. With the advance in javascript and browser support, we have many powerful chart components out there based on Javascript. Now with the help of javascript frameworks, we can create charts in just a few lines of code.
Continue reading »
Richard Wong 18 August, 2008

When it comes to cross browsers vector graphics, there have always been debate about whether to use SVG/VML or Canvas/VML. Even if you made the decision, you still need to know how to implement them.
Now Raphaël is aiming to simplify development with vector graphics across browsers. It provides us with a simple adapter to work with vector graphics using SVG/VML.
Below is an example of the basic:
// Creates canvas 320 × 200 at 10, 50
var paper = Raphael(10, 50, 320, 200);
// Creates circle at x = 50, y = 40, with radius 10
var circle = paper.circle(50, 40, 10);
// Sets the fill attribute of the circle to red (#f00)
circle.attr("fill", "#f00");
// Sets the stroke attribute of the circle to white (#fff)
circle.attr("stroke", "#fff");
If you want to create your own specific chart or image crop-n-rotate widget, you can simply achieve it with this library.
Raphaël – javascript library
Richard Wong 17 August, 2008
When it comes to pop-up windows, people don’t really associate them to being accessible and they are some what annoying. But sometimes it is necessary for whatever reason.
If you think about it, a link that opens up a new window is one of the most simple thing you can do with javascript & HTML. There is no reason for it to be ugly.
This is the old fashion and totally wrong way of doing it:
<a href="javascript:window.open(http://www.popup.com/);">Popup link</a>
Why? The a tag is not linking to a link but instead it is running some javscript. That means the whole meaning of the link is gone.
Here is what an accessible and clean link looks like:
<a href="http://www.popup.com" rel="pop-up">Open a Popup</a>
In this version, there is no inline javascript which is always a good pratice to separate HTML and javascript. It keeps everything simple and clean. The addition of the rel attribute is used to describe the relationship between the current page and the href destination of the anchor.
Now we have this beautiful HTML link. It is time to do some magic with javascript and jQuery.
( function popup() {
$( document ).ready( function() {
$("a[rel='pop-up']").click(function () {
var features = "height=700,width=800,scrollTo,resizable=1,scrollbars=1,location=0";
newwindow=window.open(this.href, 'Popup', features);
return false;
});
} );
}() );
This is a very simple javascript using jQuery. Basically, the code is looking for all the links with the rel attribute and assign a onclick event to open the pop-up window. Of course, there are different ways to abstract the above code to make it more flexible. But the idea is to have beautiful pop-up link and have the javascript to do the work later.
Richard Wong 8 July, 2008
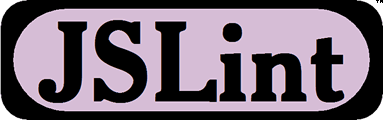
I have recently came across this interesting and useful Javascript tool called JSLint. You feed in your javascript code and JSLint will scan through and looks for problems in your code.
You might ask what is the different between this and debugging using Firebug? Well, although it is only a syntax checker and validator, it is much more stricter and follows proper Code Conventions. In other word, it will tell you errors you normally won’t get.
As you know, Javascript sometimes allows code to be implemented in a sloppy way which could be very troublesome for large complex projects. So I have been using this tool as a reference point to tighten up my javascript code.
So go and paste your code at JSLint and see how your code is doing. But I must warning you, it might hurt your feeling!
Source: JSLint